Anki Books is a flashcards/note-taking app inspired by Anki and my vision of wanting to build a collaborative platform for making Anki flashcards, with the twist that the flashcards would be inside online "books" that learners could study before downloading the flashcards. It is free and open source: Anki Books source code
Open user registration for Anki Books will not be possible for the time being, but I made public a few of my "books" that I think some people might find interesting:
Open user registration for Anki Books will not be possible for the time being, but I made public a few of my "books" that I think some people might find interesting:
Free Anki books:
- Notes on "The Linux Command Line"
- Notes on "Version Control with Git"
- Notes on "Atomic Habits"
- Notes on "The Courage To Be Disliked"
- Notes on "Code Craft"
- .NET notes
- Rough draft of when I tried to write a book
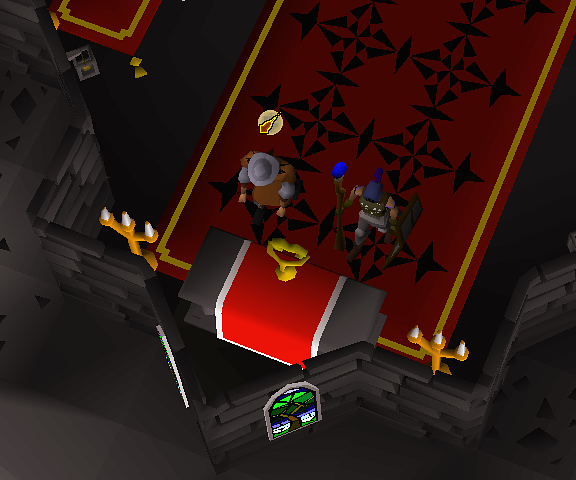
Links for me (do not click these unless your name is Kyle):